What Does ‘def’ Mean in Python?: Definition with Examples
Imagine you are the bakery owner, and every time a customer comes in, you welcome them by calling their name. Instead of repeating the same message for all customers, you can define a function in Python to handle this task efficiently.
In Python, functions are powerful tools for software construction, permitting Python function definitions that allow code reuse for specific tasks. Using functions, you can create a def function in Python, where a customer’s name is passed as an argument, and the lambda functions(nameless function) can also be used.
They enable us to pack code blocks into something that can be reused, thereby rendering programs more readable, writable and maintainable.
Mastering def in Python meaning not only simplifies coding but also improves program structure. In this article, we will explore what does def do in Python, its syntax, and practical examples.
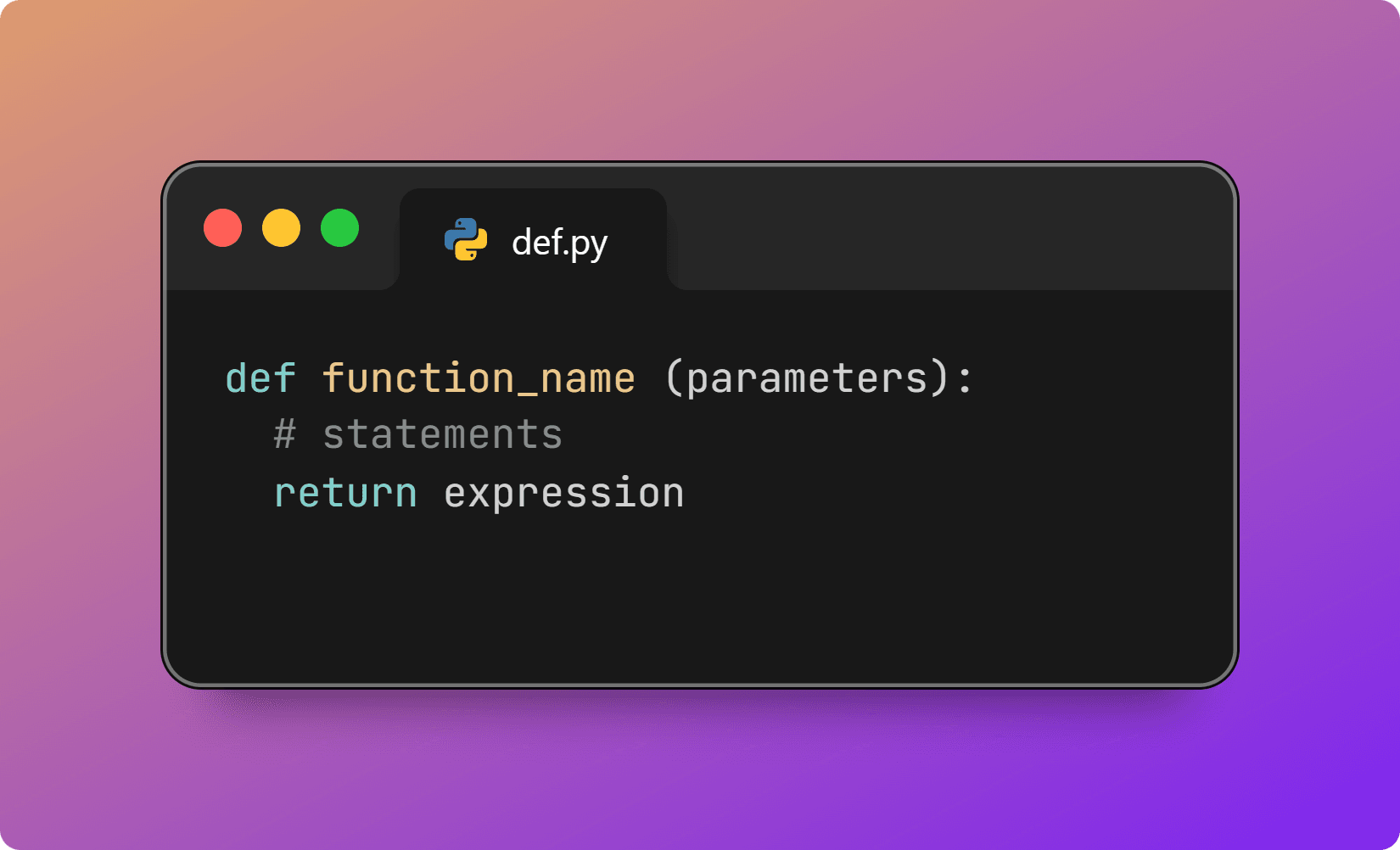
What Does def Do in Python Programming?
The def keyword is a basic syntax, which is very useful tool that serves as the backbone of function customization. Developers use it to mark the beginning of a function definition, containing collaborative code within a reusable block.
Using def function in Python improves code organization, making Python programs more structured and easier to debug and scale. Functions help break tasks into smaller, manageable units, reducing redundancy and improving clarity.
The def keyword, also known as "define" has a critical function in the implementation of all the arguments to functions that are defined inside, carry out a specific task in a python program.
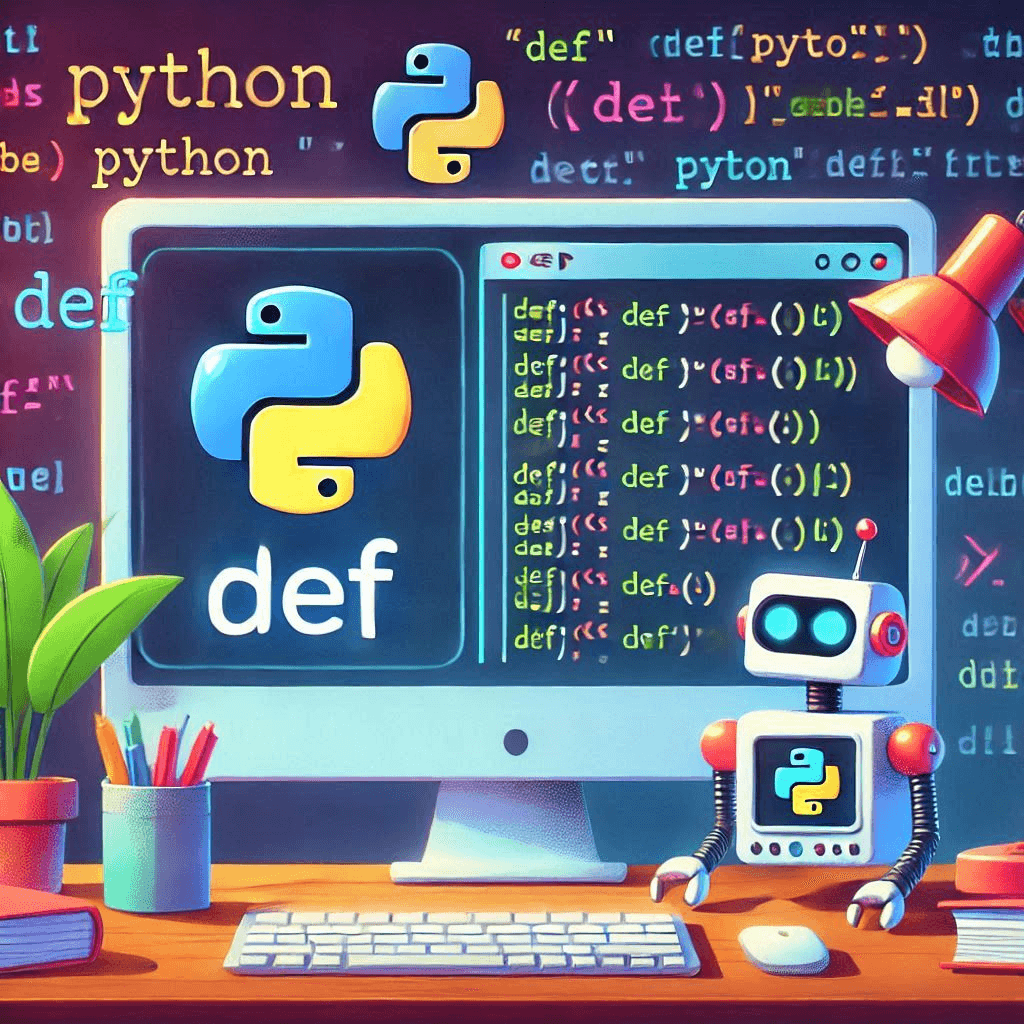
Functions can have variable parameters, providing a degree of flexibility and dynamic behaviour. These variable parameters and arguments are entered in parentheses and allow the function to operate on several inputs, including positional arguments, variable, or default arguments.
The Python function defines the function structure. The function body forms the core, containing arguments, nested logic, and function calls. This modular structure improves Python program definition, enhancing reusability. Using def in Python allows developers to write a function in Python that handles specific tasks efficiently.
Example:
The following example calculates the sum of two numbers:
def add_numbers(a, b): return a + b
In this case, the function add_numbers accepts two inputs a and b, and gives the sum of a and b as output.
Defining Functions in Python: Examples for Beginners
Let's explore practical examples of def function in Python. Simple functions and built-in functions introduce more complexity, including parameters, default values, keyword arguments, and return statements.
Let’s understand python function definitions with examples to see how functions work in real programs.
Example 1: A Basic Function Without Parameters
def greet(): print("Hello, World")
This function( greet()) doesn’t take any arguments. When greet() function is called, it simply prints Hello, World
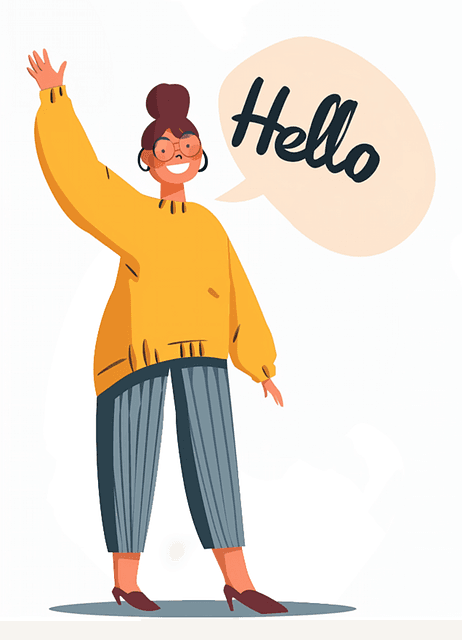
Example 2: A Function With Parameters
def multiply(x, y): return x * y
Here, the multiply function takes two parameters x and y, and the function returns their product. Parameters make functions more dynamic and versatile.
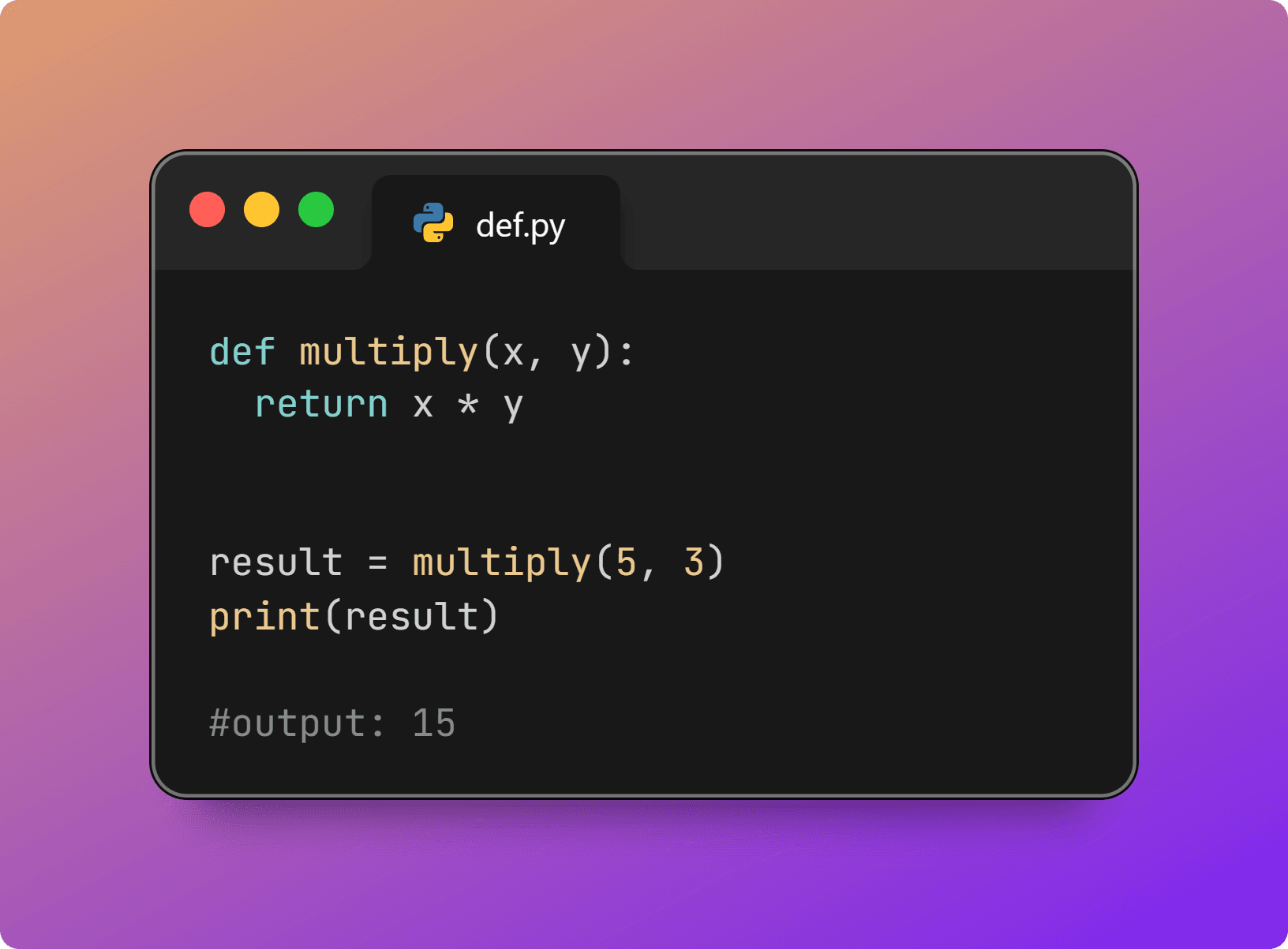
Example 3: A Function With Default Arguments
def greet_friend(name="Ravi"): print(f"Hello, {name}!")
The greet_friend function in the above example has a default first argument, a string named "Ravi". If there is no argument while calling the function, it will use the string as default value.
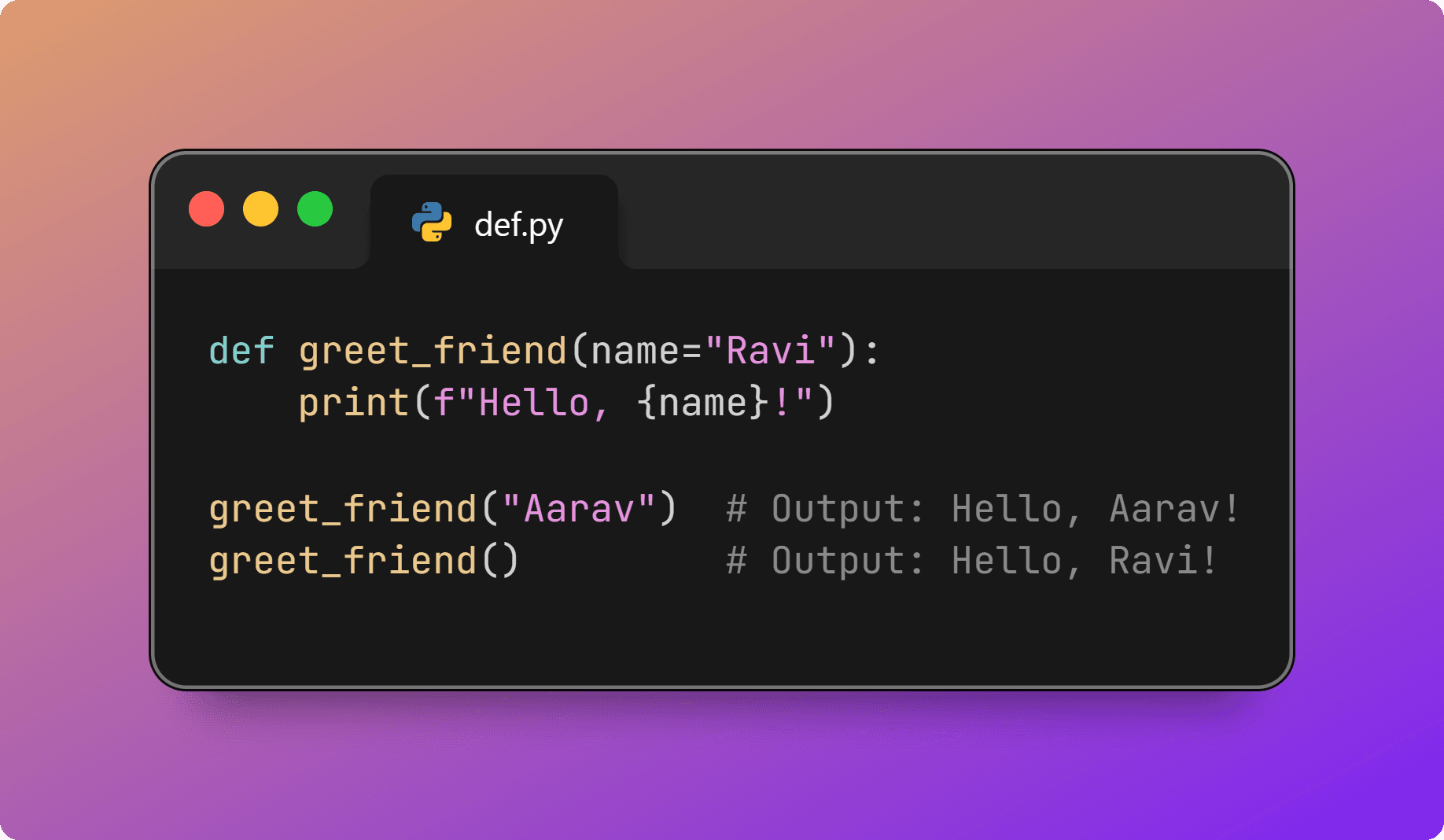
Breaking Down the Syntax of def in Python
To use recursive functions or normal Python function, it is necessary to master the syntax. The def keyword in Python is central to creating functions. Below is a detailed description of the def syntax and used in the definition of the function in Python.
Syntax Template:
def function_name(parameters): # code block return value
Key Components
def: def keyword is used at the beginning of a function.
Function name: Use the relevant function name, using Python's naming conventions (snake_case).
Parameters: These are optional and are enclosed in parentheses, parameters enable you to provide input into the function for processing.
Colon (:): It's the end of function header and is used to introduce the body of the function.
Indentation: Very important in python to specify the code section inside the function. This is unique to python and helps keep the code clean and organized.
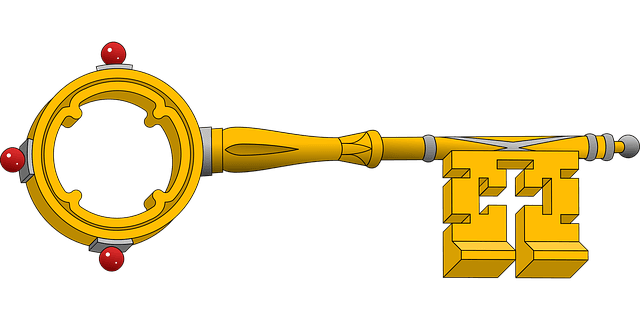
Best Practices for Writing Functions in Python
Use Descriptive Function Names
A clear name improves readability. For example, use calculate_area instead of a vague name like area.def calculate_area(length, width): """Calculate the area of a rectangle.""" return length * width
Focus on a single task
All functions serve a single purpose and do so properly. This makes your code modular and easier to debug
Include comments or docstrings
Just comment or use docstrings to describe what the function is supposed to do. This is helpful for others to understand your code.
Use parameters instead of hardcoding values
Avoid embedding fixed values. Instead, use parameters to make functions flexible.
Keep functions small
Large functions are hard to read and maintain. Break them into smaller, reusable chunks if necessary.
Conclusion
Functions, defined by the keyword def in Python, allow programmers to break down complex tasks into smaller, manageable chunks that enhance the readability, productivity, and effectiveness of code. Python function definitions serve as an interface to specify functions that organize and facilitate code reusability.
Whether you're adding functions in Python to a simple script or a large-scale project, the correct use of def syntax guarantees that your code remains structured, maintainable, and efficient. Experiment with different approaches and integrate best practices into your own functions and projects.
Python - FAQs
How can I define a function in Python?
In def Python, creating a function definition begins with the word "def" keyword. This keyword indicates the beginning of a function definition. After def, generate a descriptive and call the function by name, followed by parentheses surrounding optional parameters. End the line with a colon(:) Below the line indent all the code within the function body performing the intended task.
Example:
def greet_user(): print("Hello, World")
In this example:
def introduces the function definition.
greet_user is the function name.
The indented code forms the function body
What does def stand for in Python, and why is it important?
def stands for "define". It is a core functionality of programming by which developers can implement user-customized functions. Functions are essential in decomposing code into smaller units, with a higher degree in modularity and reusability.
Example:
def add_numbers(a, b): return a + b
In this case, def defines a function named add_numbers() that takes two positional arguments (a and b). The function body then returns the sum of the two arguments. Functions like this one, allow python programs to avoid redundancy, improve maintainability, and provide a reusable block of code that can be called multiple times, making the program more efficient.
How do I use parameters in a Python function definition?
Parameters are placeholders for data passed into a function. They are given in parentheses at the top, called a function by definition, and used in the function body to perform actions using actual values of their dynamically changing arguments.
Example:
def greet(name): print(f"Hello, {name}!")
Here, the argument is the name, and the function can be applied to any other object as an argument is the object passed to the function call.
How does def simplify writing Python programs?
In Python, the def keyword is used to provide convenience in programming because it encourages code reusability by generating functions. If you define a function using def, it contains a piece of logic in a reusable unit.
This helps reduce duplication since repetition of similar code within a program is no longer required. However, you can just call the function whenever needed, increasing maintainability and efficiency.
What are the best practices for writing Python functions using the def keyword?
To ensure your Python functions are practical and maintainable, follow these best practices:
Use descriptive names: Choose meaningful names for functions that indicate their purpose (e.g., calculate_area, fetch_data).
Keep functions focused: Ensure each function performs a single, well-defined task. This makes functions more straightforward to understand and test.
Include comments/docstrings: Include comments and docstrings describing what the function does, its parameters, and its return values. This improves the readability and usability of the code.
Maintain code readability: Maintain proper indentation and follow Python's PEP 8 style guide for consistency. This ensures that others can read and understand your code easily.
Best Practice | Description | Example |
Use descriptive names | Choose clear, meaningful names for functions that indicate purpose | ![]() |
Keep functions focused | Ensure each function performs a single, well-defined task. This makes functions easier to understand. | ![]() |
Include comments/docstrings | Provide comments or docstrings to describe what the function does, its parameters, and its return values. | ![]() |
Ensure code readability | Maintain proper indentation | ![]() |
What happens if I don’t use the def keyword correctly in Python?
If you don't use the def keyword in Python, you will encounter a syntax error, which will stop your program from running correctly. The def keyword is to define a function, and any errors in its syntax, such as the lack of colons or incorrect indentation, may result in a runtime error.
Errors in this format can invalidate Python function definitions and halt program execution. It's important to observe the right syntax for correctly defining the Python function so that it is correct and the Python function behaves accordingly.
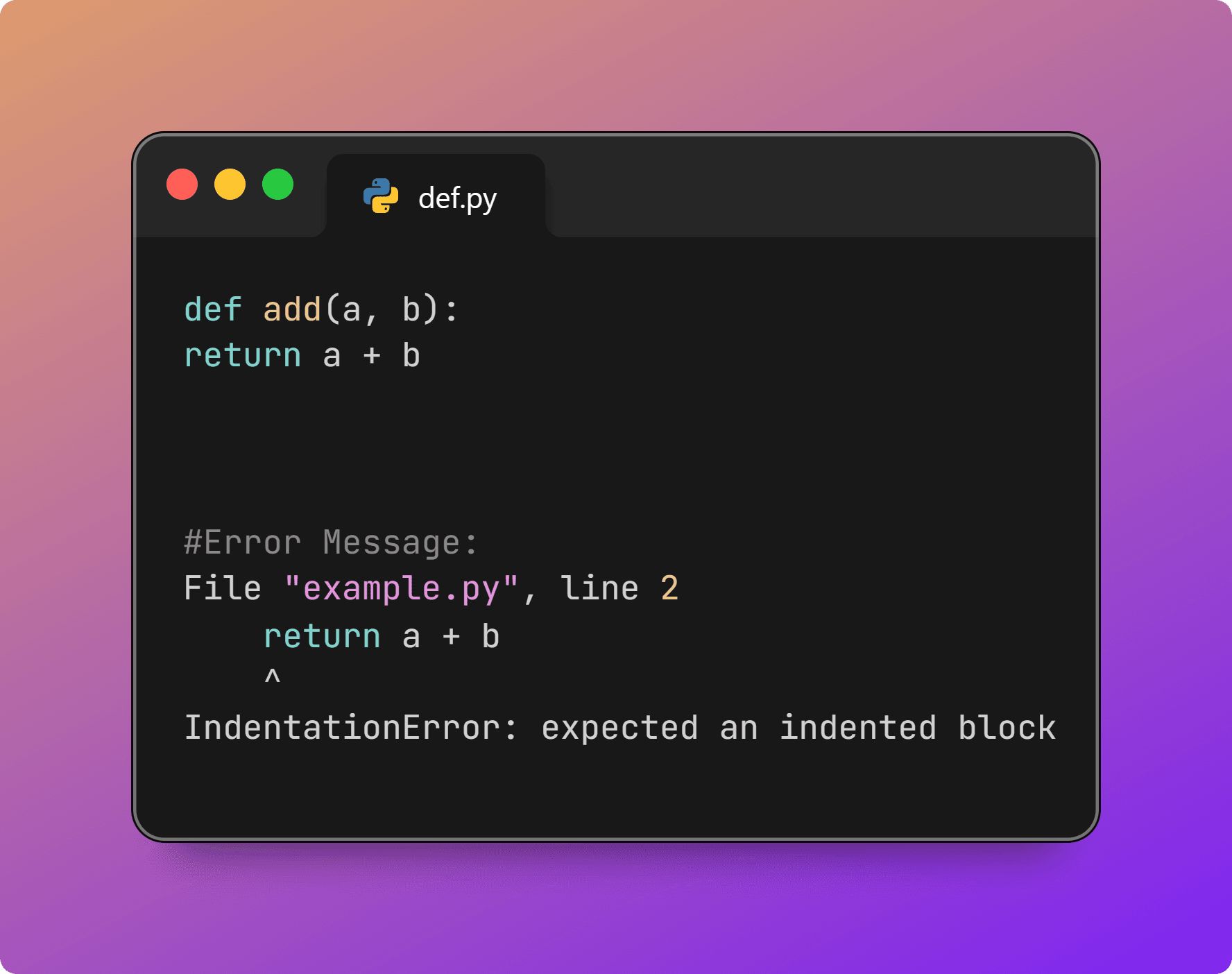
What are common errors when defining functions in Python
When def is used improperly in Python, it can cause syntax errors to block the execution of your program. Some common mistakes include:
Forgetting the colon (:) after the function definition.
Incorrect or inconsistent indentation in the function body.
Not using parentheses correctly in function calls or definitions.
Incorrectly using default arguments without providing proper values.
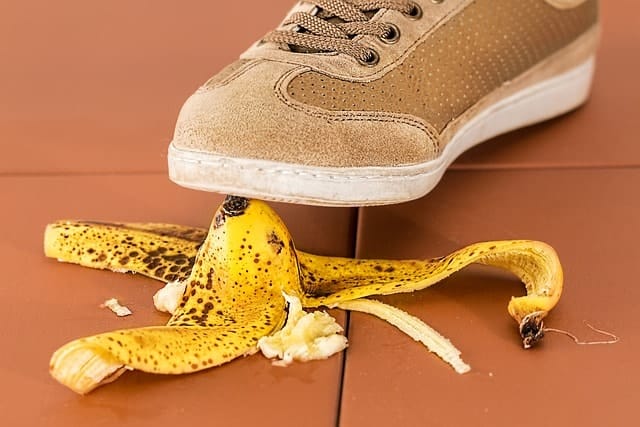