Mastering Basic JavaScript Classes: The First Step for Young Coders
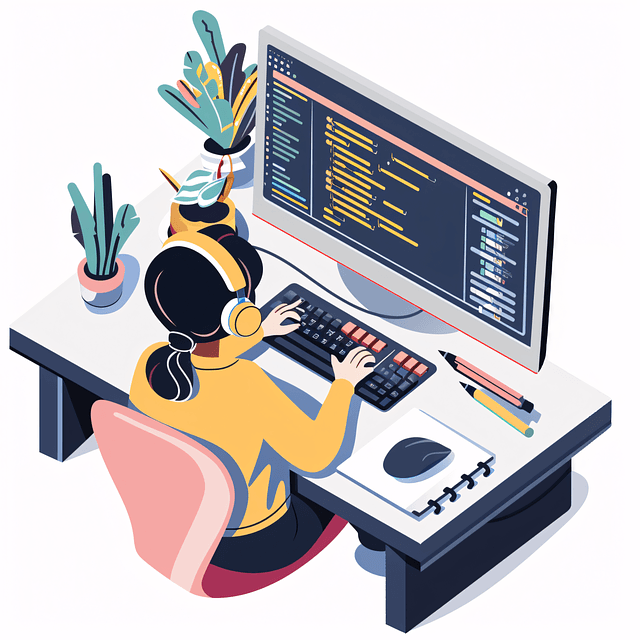
JavaScript classes have changed how programmers build software. They add structure, efficiency and reusability to code. They are the basis of object-oriented programming (OOP), a paradigm fundamental to making scalable web applications, interactive games, and enterprise-grade software. OOP is key to building scalable solutions, from web apps to dynamic games. OOP breaks complex tasks into smaller, manageable pieces.
JavaScript classes are an excellent start for young coders. They build strong programming skills, simplify challenging concepts like data organisation and behaviour design, and use intuitive syntax. Learning these classes early boosts problem-solving and logic. It also lays a solid foundation for using advanced frameworks like React later.
The basic JavaScript class syntax forms the base. It's a starting point to build your skills for serious coding. It allows you to explore advanced topics and contribute to real projects.
What Is JavaScript Class?
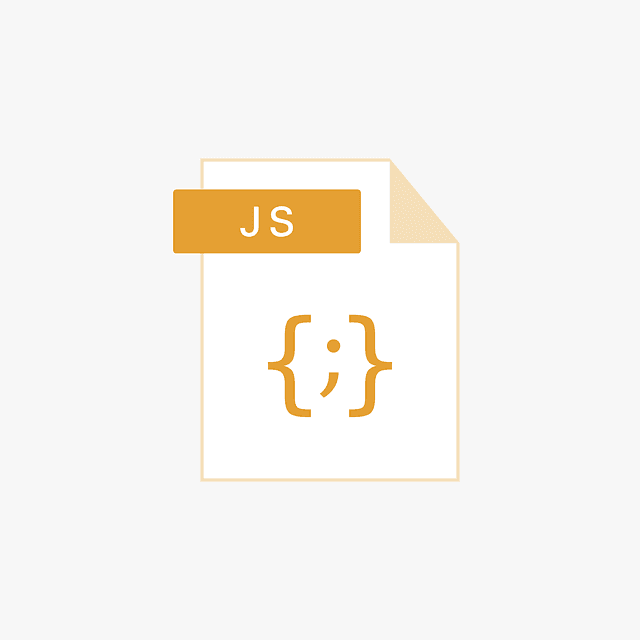
What Are JavaScript Classes?
JavaScript Classes in most programming languages can structure your code and enable re-usability. For young coders, classes in JavaScript are the first step toward learning object-oriented programming, a widely used way of building apps and games.
JavaScript classes are basic templates for creating objects in other programming languages. Think class as a recipe. You have a recipe for cookies, a class point and every time you bake some of that class myclass recipe, it’s an object.
Why Should You Learn About JavaScript Classes?
Organizing Code Easily: Classes keep related information and actions together.
For example, if you are making a game, you can make a Player class to hold the player's name, score and something like jump or run.
Reusable Blueprints: You can use a class as many times as you want once you build it without having to create everything from the beginning
Real-World Applications:
Games: Use classes to build characters, levels, and items.
Web Apps: JavaScript classes easily manage users, tasks, or chat messages.
Mobile Apps: Organize features like buttons, animations, or settings using classes.
How a JavaScript Class Works
class Dog { constructor(name, breed) { this.name = name; this.breed = breed; } bark() { return `${this.name} says Woof`; } } // Creating a dog objectconst myDog = new Dog("Buddy", "Golden Retriever"); console.log(myDog.bark()); // Output: Buddy says Woof
In this example:
Class Dog is like a template.
When creating an object, the constructor initializes the name and breed of the dog.
The bark() method tells the dog what to say.
Using JavaScript classes, you can build everything from a list of homework assignments to a scoreboard for your favourite game.
Understanding JavaScript Class Syntax
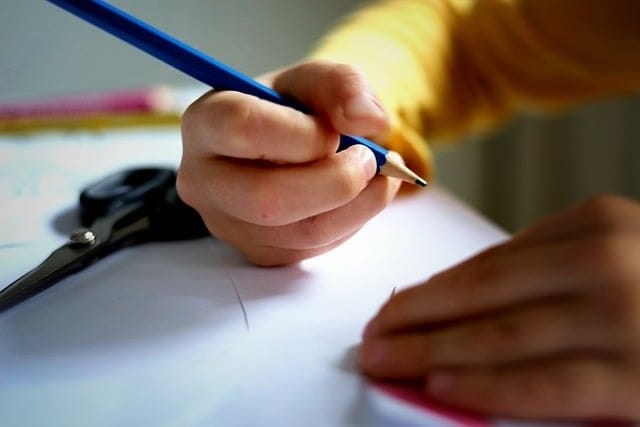
When we talk about JavaScript classes, we use syntax for class members' object methods to simplify the many objects created in object-oriented programming. This part explains the input component in the class definition, followed by examples for simple exercises.
1. Class Declaration
A class declaration in JavaScript starts with the class keyword followed by the class name, which is normally capitalised. A special function named the constructor is defined within the class and is used to initialise object properties when you create a new object. This makes it a great fit for structured and reusable code. It allows developers to encapsulate data and behaviour in class expressions, including methods typing to properties in the same class expression.
class Person { constructor(name, age) { this.name = name; this.age = age; } greet() { return `Hi, I am ${this.name} and I am ${this.age} years old.`; } } const person1 = new Person("Ajay", 16); console.log(person1.greet());
Key Points:
A person is a class declaration.
The constructor method initializes the object properties, such as name and age.
The greet function is an example of a class method that defines class behaviour.
2. Constructor Method
The constructor method is called automatically when a new class instance is created. It’s the constructor method used to set up the initial values, such as object methods.
class Dog { constructor(breed) { this.breed = breed; } } const dog1 = new Dog("Labrador"); console.log(dog1.breed); // Output: Labrador
Key Points:
Class constructor: Sets initial values during class instance creation.
Constructor name: Matches the class name and is used to initialise object data.
3. Class Methods and Static Methods
Class methods define actions related to the object.
Static methods belong to the class itself, not individual objects.
class MathAddition { static add(a, b) { return a + b; } } console.log(MathAddition.add(3, 7)); // Output: 10
Note: Use static methods for utility functions and class methods for behaviours tied to instances of function property and static method.
Comparing JavaScript Class Syntax with Function-Based Approaches
In JavaScript, classes are a shorthand for existing prototypes. They use constructor functions for prototypical inheritance. Classes provide a better way to create and manage objects.
Function-Based Approach
JavaScript classes were based on constructor functions, which defined objects and their properties before classes. To make this more efficient, methods class fields were automatically added to class, and function declarations were used in the prototype.
function Person(name, age) { this.name = name; this.age = age; } Person.prototype.greet = function () { return `Hi, I'm ${this.name}, and I'm ${this.age} years old.`; }; const person1 = new Person("Arun", 25); console.log(person1.greet());
The Person function acts as a constructor function.
Person.prototype.greet adds a method, but the syntax can be verbose.
This approach relies heavily on the prototype chain for method sharing.
Class-Based Approach
The class syntax added in ES6(ECMAScript 2015) helps us easily create object-derived classes and methods. The class declarations, entire constructor, and methods are wrapped in a single readable structure.
class Person { constructor(name, age) { this.name = name; this.age = age; } greet() { return `Hi, I am ${this.name}, and I am ${this.age} years old.`; } } const person1 = new Person("Ravi", 20); console.log(person1.greet()); // output: Hi, I am Ravi, and I am 20 years old.
Advantages of Class Syntax:
The class declaration also will combine the constructor and methods into a single code block.
Class methods (like greetings) are defined inside the class body.
More readable and maintainable than function-based alternatives.
Key Differences
Feature | Function-based Approach | Class based Approach |
Syntax | Relies on function keywords and prototypes. | Uses class keywords for clear structure. |
Method Definition | Added to prototype manually. | Defined directly in the class body. |
Inheritance | Achieved with Object.create() or prototypes. | Simplified with extended keyword. |
Encapsulation | Scattered across function and prototype. | Unified in a single class definition. |
What are the Benefits of Learning JavaScript Class?
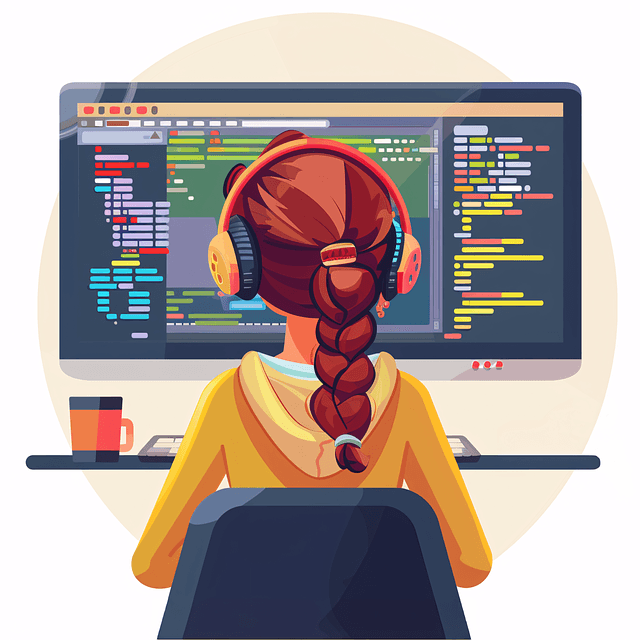
JavaScript classes are a great way to build reusable and modular code, a must-know skill for young developers.
If you know the class keyword, how to create a class, etc, you can group similar object properties and methods. This means that once you write a Person class, you do not have to write the same code whenever you want a new Person. This keeps your code cleaner and helps prevent mistakes, making programming more enjoyable.
Apart from simplifying coding, JavaScript classes develop your problem-solving capabilities. With constructor functions and class methods, you bring an object-oriented mindset to how the different pieces interact. As you approach more advanced programming challenges, this thinking is beneficial.
JavaScript classes are essential for any developer in tech, especially when working with frameworks like React and Angular. JavaScript classes were introduced in ECMAScript 2015 (ES6), allowing us to create reusable code by encapsulating our data and behaviour in a single template. It enables developers to develop properties and methods with the class keyword and constructor method, hence instantiating multiple objects from a single class without redundancy.
Types of JavaScript Classes
In JavaScript, classes can be categorized into different types based on their naming and usage. They are :
Default Classes
A default class is a JavaScript class declaration without an explicit name. These are used when exporting a single class from a file or module.
Example:
export default class { constructor(name) { this.name = name; } display() { console.log(`Name: ${this.name}`); } }
Use Case:
Default classes are helpful when you don’t need to identify the class elsewhere in the code, and they are commonly used in module exports.
Named Classes
Named classes have a defined name that can be referenced within the scope of methods defined the name of the program.
Example:
class Person { constructor(name, age) { this.name = name; this.age = age; } introduce() { console.log(`Hi, I'm ${this.name} and I'm ${this.age} years old.`); } }
Use Case:
Named classes, like the Person class, are ideal for readability and debugging since their name appears in stack traces following the code name.
Anonymous Classes
Anonymous classes are defined without a name and are typically assigned to variables.
Example:
const Animal = class { constructor(type) { this.type = type; } describe() { console.log(`This is a ${this.type}.`); } };
Use Case:
Anonymous classes are used for dynamic behaviour or when the name of the regular function isn’t necessary.
Essential Things to Know about JavaScript Classes
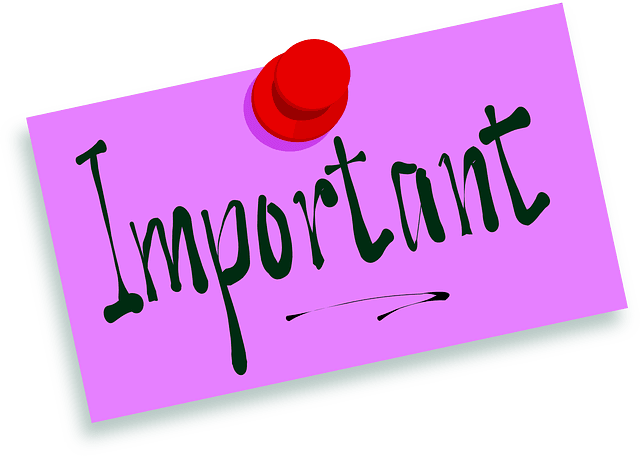
JavaScript classes are blueprints used to create objects with similar behaviours. As a beginner, you must learn about classes. It's the first step to making your code organised and easier to use. Let's find some common mistakes and best practices.
Common Mistakes Kids Often Make
Using Classes When Not Needed
If you need a tool to fix a small problem, you bring a big toolbox with a ton of tools just in case. That’s the effect of using the objects created in a JavaScript class for tasks that appear simple. So, constructor functions or objects are all you need.
Forgetting to Name Classes Correctly
Always name your classes in PascalCase. It means the first letter of every word is capitalized.
For example:
✅ PersonClass
❌ person class
Confusion with Inheritance
When you use the extends keyword to make a new class inherit from another, don’t mix up the same methods or properties. If a class derived extends the base, ensure the new class adds value and doesn’t copy everything.
Best Tips for Writing Great JavaScript Classes
Pick Clear Class Names: Class names such as AnimalClass or PersonClass can quickly tell what the class is doing.
Keep Methods Inside the Class: Move all methods inside the class body for readability.
Use Static Methods for Class-Wide Actions: Use a static method for things not class instance/object dependents.
Example:
class Calculator { static add(a, b) { return a + b; } } console.log(Calculator.add(3, 4)); // Output: 7
How JavaScript handles inheritance, encapsulation, and prototyping through classes
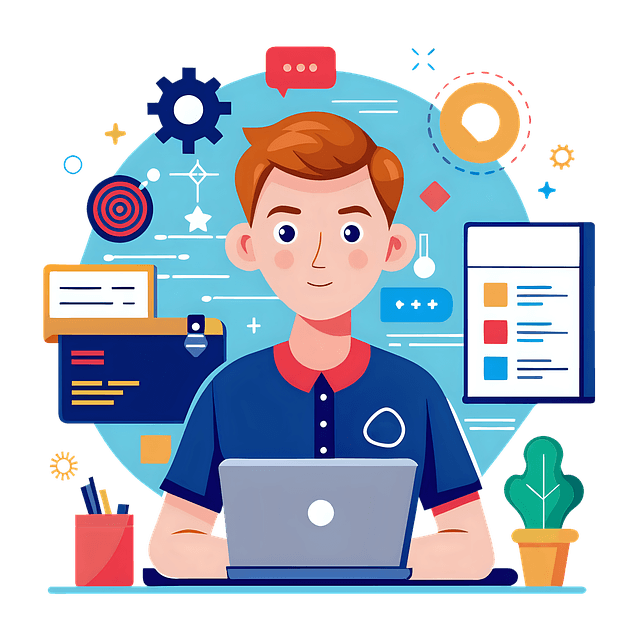
Inheritance
Imagine passing down skills from parent to child. If you have a class called Dog Extends Animal, the Dog class can do everything an Animal class point does and some special tricks of its own.
Example:
class Animal { eat() { console.log('Yum'); } } class Dog extends Animal { bark() { console.log('Woof'); } } const dog = new Dog(); dog.eat(); // Output: Yum dog.bark(); // Output: Woof
Encapsulation
Imagine your phone has apps, but you can’t see how the phone’s system works. Encapsulation in JavaScript classes hides certain details while letting you use others.
Example:
class SecretBox { #secret = 'hidden treasure'; revealSecret() { return this.#secret; } } const box = new SecretBox(); console.log(box.revealSecret()); // Output: hidden treasure
Prototyping
JavaScript uses the prototype chain to implement prototyping, allowing objects instantiated from a class or constructor function to share the methods and properties the constructor defines. A new instance will also inherit methods invoked by the prototype of that class when created with the new operator. This means they don't need to repeat methods for every object, optimising the code.
Why Should You Learn JavaScript Classes?
Organize your code: Use JavaScript classes to group related actions and data. This makes your code cleaner and easier to manage.
Avoid repetition: Use JavaScript classes to create templates. You can then reuse them instead of writing the same logic repeatedly. This saves time and reduces errors.
Master popular frameworks: Frameworks like React and Angular are built on JavaScript classes. Learning them now means you will be ready to dive into these powerful tools without feeling lost.
Build real-world projects: Whether it's a simple app, an advanced game, or a dynamic website, classes make your code more professional and maintainable.
Creating Your First JavaScript Class: A Beginner-Friendly Guide
Step 1: What’s a JavaScript Class?
Think of a JavaScript class as a recipe. It tells you what ingredients (properties) and steps (methods) are needed to make something like a "Student" or "Car."
Example:
class Car { constructor(brand, model) { this.brand = brand; // Car's brand this.model = model; // Car's model } honk() { console.log("Beep beep!"); } }
Step 2: Building Objects from a Class
Once you have the recipe, you can create as many cars (or objects) as you like.
let myCar = new Car("Tesla", "Model 3"); console.log(myCar.brand); // Output: Tesla myCar.honk(); // Output: Beep beep!
This is called "creating objects" from code inside a class
Step 3: Understanding the Constructor
The constructor is like setting up the toy parts. It runs automatically when you create a new object. For example:
let anotherCar = new Car("Ford", "Mustang"); console.log(anotherCar.model); // Output: Mustang
The constructor method helps each car (object) have its constructor name and own unique details.
Step 4: What Are Properties?
Properties are the things that make your objects unique, like:
A student’s name and grade.
A car’s colour and speed.
For instance, in a car example, brand and model are properties.
Step 5: Using Methods for Action
Methods are like buttons on your toy. When you press one, something happens. In the car example, the honk method makes the car beep.
class Dog { bark() { console.log("Woof!"); } } let myDog = new Dog(); myDog.bark(); // Output: Woof!
Key Concepts: Constructor, Properties, and Methods
Constructor
The constructor is a special method of a class in JavaScript that is used to initialise the objects. It is invoked automatically whenever an object of the class is created. It is used to set up initial values of properties.
Example:
class Person { constructor(name, age) { this.name = name; // Property this.age = age; // Property } }const person1 = new Person('John', 30);console.log(person1.name); // Output: Johnconsole.log(person1.age); // Output: 30
In this example, the constructor sets the name and age of the class person as properties when creating a Person object. The constructor simplifies object creation and ensures that you provide initial values.
Properties
Properties describe the characteristics or data about an object. They are declared in the class definition and can be initialized by a constructor. These attributes enable a class to represent and control data.
class Dog { constructor(breed, color) { this.breed = breed; // Property this.color = color; // Property } }const myDog = new Dog('Labrador', 'Black');console.log(myDog.breed); // Output: Labradorconsole.log(myDog.color); // Output: Black
Here, breed and color are properties of the Dog class. They store the dog's characteristics.
Methods
Methods are actions or behaviours that an object can perform. They are defined inside the class body and can manipulate or use the class properties. Class methods include both instance methods and static methods.
Instance Method Example:
class Car { constructor(model, year) { this.model = model; this.year = year; } startEngine() { // Method console.log(`${this.model} is starting.`); } }const myCar = new Car('Toyota', 2022);myCar.startEngine(); // Output: Toyota is starting.
The startEngine method performs an action using the class's properties.
Static Method Example:
Static methods are called on the class itself, not on instances of objects created in the class.
class MathAdd { static add(a, b) { return a + b; } }console.log(MathAdd.add(5, 3)); // Output: 8
In this case, add is a static method of the MathAdd class, performing a calculation without needing an object instance.
JavaScript Classes - FAQs
How Do Classes Work in JavaScript?
JavaScript classes are templates for creating objects. They encapsulate data (properties) and behaviour (methods). A class is defined using the class keyword, and you can create new instances using the new operator. The constructor function executes without manual intervention when a new object is created. It initializes the object's properties.
Do I Need To Know HTML And CSS Before Learning JavaScript?
It's not mandatory, but knowing HTML and CSS helps. It will help you understand how JavaScript interacts with web pages. JavaScript enhances HTML and CSS by adding interactivity, making websites dynamic. Starting with basic HTML and CSS provides a solid foundation for learning JavaScript.
How long does it take to learn JavaScript?
It can take 2 to 6 months to learn JavaScript. It depends on your experience and daily practice. Beginners may need more time to grasp the syntax. But, those with programming experience can learn faster. Consistent practice and working on projects speed up the learning process.
What is the JavaScript class method?
A class method in JavaScript is a function defined inside a class. It operates on class instances and can access the object’s properties using this. Unlike regular functions, class methods tie themselves to the object they belong to.
How are methods different from properties in a JavaScript class?
Properties store data, while methods define actions. A Car class might have a model property and a startEngine method. Properties define an object’s state, whereas methods define behaviour.
Why is learning JavaScript classes important for young coders?
Learning JavaScript classes enhances structured thinking, which is essential for problem-solving. It introduces object-oriented programming, which helps transition to complex languages like Python or Java.
Is JavaScript easier than Python?
Both are suitable for beginners. However, Python is often considered easier due to its simple syntax. But JavaScript is essential for web development. It depends on your goals. Web development favours JavaScript. Data science leans toward Python.